Playlist Implementation Using VdoCipher SDK
This guide will walk you through the implementation of a playlist feature in an Android app using the VdoCipher SDK. The playlist allows users to play a series of videos one after another, with the ability to switch between them using a RecyclerView.
Implementation Overview
We'll walk through creating a video playlist using VdoPlayerUIFragment. The playlist will allow users to browse and play videos, where each video will automatically switch to the next one when it ends. Additionally, when the playlist reaches the last video, it will loop back to the start and continue playing from the first video again.
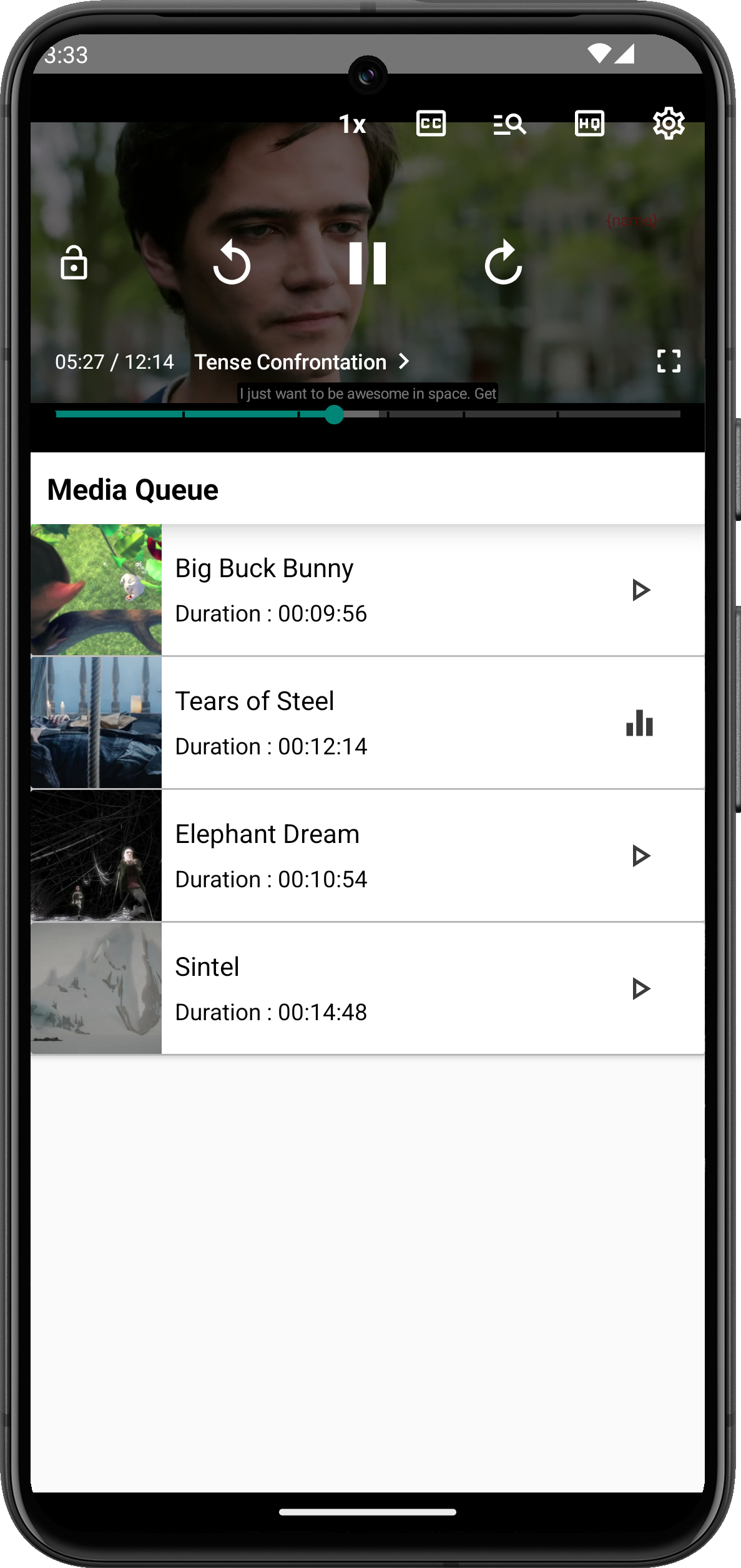
Step-by-Step Implementation
1. Setup VdoCipher SDK
Ensure that the VdoCipher SDK is integrated into your project. Follow the official integration guide if you haven't done so already.
2. Activity Setup
In this section, we set up the VdoPlayerUIFragment and RecyclerView to manage video playback and display the list of videos in the playlist.
- Java
- Kotlin
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_vdo_playlist);
// Setup Player Fragment for video playback
newFragment = (VdoPlayerUIFragment) getSupportFragmentManager().findFragmentById(R.id.vdo_player_ui_fragment);
// Setup RecyclerView for displaying the playlist
RecyclerView recyclerView = findViewById(R.id.recycler_view);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.addItemDecoration(new DividerItemDecoration(recyclerView.getContext(), LinearLayoutManager.VERTICAL));
// Set adapter to manage playlist items
videoAdapter = new VideoAdapter(this);
recyclerView.setAdapter(videoAdapter);
// Load the playlist and initialize the player
loadPlaylist();
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_vdo_playlist)
// Setup Player Fragment for video playback
newFragment = supportFragmentManager.findFragmentById(R.id.vdo_player_ui_fragment) as VdoPlayerUIFragment
// Setup RecyclerView for displaying the playlist
val recyclerView = findViewById<RecyclerView>(R.id.recycler_view)
recyclerView.layoutManager = LinearLayoutManager(this)
recyclerView.addItemDecoration(DividerItemDecoration(recyclerView.context, LinearLayoutManager.VERTICAL))
// Set adapter to manage playlist items
videoAdapter = VideoAdapter(this)
recyclerView.adapter = videoAdapter
// Load the playlist and initialize the player
loadPlaylist()
}
3. Load Playlist & Initialize Player
Here we load the list of videos into the playlist and initialize the player to get ready for video playback.
- Java
- Kotlin
private void loadPlaylist() {
// Add media items to the playlist
mediaItems.addAll(new PlaylistHolder().getMediaItems());
// Submit the playlist to the RecyclerView adapter
videoAdapter.submitList(new ArrayList<>(mediaItems));
// Initialize the player fragment
newFragment.initialize(initializationListener);
}
private fun loadPlaylist() {
// Add media items to the playlist
mediaItems.addAll(PlaylistHolder().getMediaItems())
// Submit the playlist to the RecyclerView adapter
videoAdapter.submitList(ArrayList(mediaItems))
// Initialize the player fragment
newFragment.initialize(initializationListener)
}
4. Play Videos
This section manages video playback. It plays the next video when one finishes and allows playing a specific video based on its position in the playlist.
- Java
- Kotlin
// Play the next video in the playlist
private void playNextVideo() {
currentMediaIndex = currentMediaIndex % mediaItems.size();
playViewAtPosition(currentMediaIndex);
currentMediaIndex++;
}
// Play video at a specific position in the playlist
private void playViewAtPosition(int position) {
currentMediaIndex = position;
loadVideo(currentMediaIndex);
videoAdapter.submitList(mediaItems);
}
// Load video based on its index in the playlist
private void loadVideo(int position) {
MediaItem mediaItem = mediaItems.get(position);
// Create initialization parameters for the video
VdoInitParams vdoInitParams = new VdoInitParams.Builder()
.setOtp(mediaItem.otp)
.setPlaybackInfo(mediaItem.playbackInfo)
.build();
// Load the video in the player
vdoPlayer.load(vdoInitParams);
}
// Play the next video in the playlist
private fun playNextVideo() {
currentMediaIndex = currentMediaIndex % mediaItems.size
playViewAtPosition(currentMediaIndex)
currentMediaIndex++
}
// Play video at a specific position in the playlist
private fun playViewAtPosition(position: Int) {
currentMediaIndex = position
loadVideo(currentMediaIndex)
videoAdapter.submitList(mediaItems)
}
// Load video based on its index in the playlist
private fun loadVideo(position: Int) {
val mediaItem = mediaItems[position]
// Create initialization parameters for the video
val vdoInitParams = VdoInitParams.Builder()
.setOtp(mediaItem.otp)
.setPlaybackInfo(mediaItem.playbackInfo)
.build()
// Load the video in the player
vdoPlayer.load(vdoInitParams)
}
5. Handle Player Events
Here we handle player events. When a video ends, the next video in the playlist is automatically played.
- Java
- Kotlin
// Handle playback events
private final VdoPlayer.PlaybackEventListener playbackListener = new VdoPlayer.PlaybackEventListener() {
....
@Override
public void onMediaEnded(VdoInitParams vdoInitParams) {
// Play the next video in the playlist when the current video ends
playNextVideo();
}
.....
};
// Handle playback events
private val playbackListener = object : VdoPlayer.PlaybackEventListener {
....
override fun onMediaEnded(vdoInitParams: VdoInitParams) {
// Play the next video in the playlist when the current video ends
playNextVideo()
}
....
}
Summary
By following this guide, you have implemented a video playlist feature using VdoPlayerUIFragment and RecyclerView. This setup allows users to interact with a list of videos, and the player automatically transitions to the next video in the playlist upon completion. The playlist also loops back to the beginning once the last video is played.
For the complete implementation, you can find the source code on Github.
Feel free to customize and expand upon this implementation to suit your application's specific needs.
If you have any questions or need further assistance, please contact us at support@vdocipher.com