Implementing Video Playlists with VdoCipher in Flutter
Overview
This guide will walk you through the implementation of a playlist feature in a Flutter app using the vdocipher_flutter package. The playlist allows users to play a series of videos one after another, with the ability to switch between them using a ListView widget.
We'll walk through creating a video playlist using the VdoPlayer widget. The playlist will allow users to browse and play videos, where each video will automatically switch to the next one when it ends. Additionally, when the playlist reaches the last video, it will loop back to the start and continue playing from the first video again.
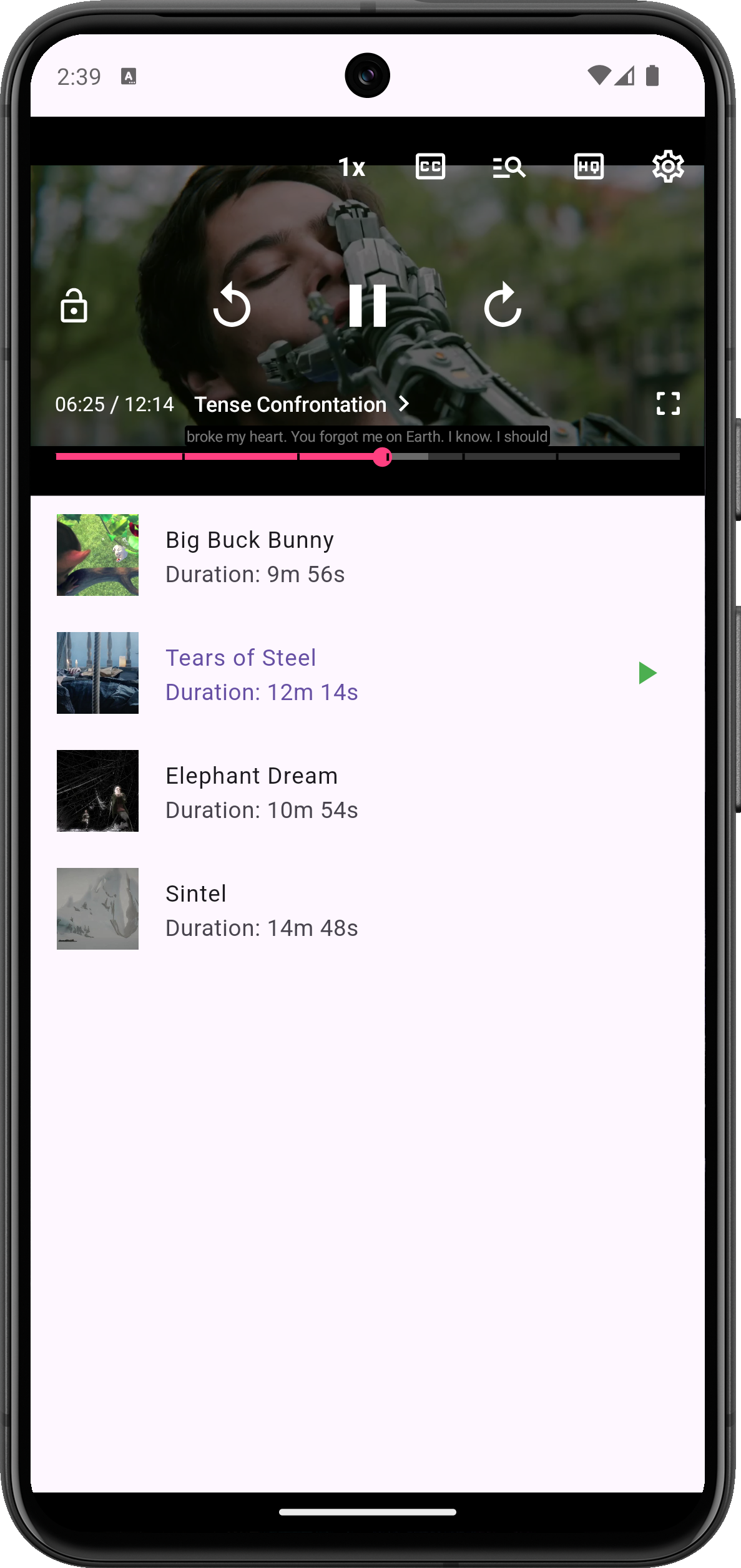
Step-by-Step Implementation
1. Setting Up the Package
Ensure that the vdocipher_flutter package is integrated into your project. Follow the official integration guide if you haven't done so already.
2. Building the Video Player Interface
Design a VdoPlaylistView
widget to display the video player and handle playback. Use the VdoPlayer
widget for video playback, and create a user interface that integrates with a PlaylistManager
to control video playback.
class VdoPlaylistView extends StatefulWidget {
const VdoPlaylistView({Key? key}) : super(key: key);
State<VdoPlaylistView> createState() => _VdoPlaylistViewState();
}
class _VdoPlaylistViewState extends State<VdoPlaylistView> {
VdoPlayerController? _controller; // Controller for VdoPlayer
final double aspectRatio = 16 / 9; // Aspect ratio for the video player
late PlaylistManager playlistManager; // Playlist manager for handling media items
void initState() {
super.initState();
playlistManager = PlaylistManager(); // Initialize PlaylistManager
}
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: Column(
children: [
// Video player widget
Flexible(
child: SizedBox(
width: MediaQuery.of(context).size.width, // Set width to screen width
height: MediaQuery.of(context).size.width / aspectRatio, // Calculate height based on aspect ratio
child: VdoPlayer(
embedInfo: playlistManager.getCurrentMediaItem().getEmbedInfo(), // Get EmbedInfo for the current media item
onPlayerCreated: _onPlayerCreated, // Callback for player creation
onError: _onVdoError, // Callback for player errors
),
),
),
// Playlist view
Flexible(
child: ListView.builder(
itemCount: playlistManager.getPlaylistLength(), // Number of items in the playlist
itemBuilder: (context, index) {
final mediaItem = playlistManager.getMediaItemAtIndex(index); // Get media item at current index
bool isPlaying = index == playlistManager.currentIndex; // Check if the media item is currently playing
return GestureDetector(
onTap: () {
playMedia(index); // Play media item on tap
},
child: ListTile(
leading: Image.network(mediaItem.thumbnailUrl), // Display thumbnail image
title: Text(mediaItem.title), // Display title
subtitle: Text("Duration: ${formatDuration(mediaItem.duration)}"), // Display duration
trailing: isPlaying ? Icon(Icons.play_arrow, color: Colors.green) : null, // Show play icon if playing
selected: isPlaying, // Highlight the currently playing item
),
);
},
),
),
],
),
),
);
}
// Callback when the VdoPlayer is created
void _onPlayerCreated(VdoPlayerController? controller) {
setState(() {
_controller = controller; // Set the controller
});
}
// Callback when there is an error in the VdoPlayer
void _onVdoError(VdoError vdoError) {
print("Error: ${vdoError.message}");
}
// Method to play a media item by index
void playMedia(int index) {
MediaItem mediaItem = playlistManager.playMediaAtIndex(index); // Get media item at index
_controller?.load(mediaItem.getEmbedInfo()); // Load media into player
}
}
3. Implementing the Playlist Manager
The PlaylistManager
class handles media playback management, including keeping track of the current media item and managing transitions between media items in a playlist.
class PlaylistManager {
List<MediaItem> _mediaItems = []; // List to hold media items
int currentIndex = 0; // Index of the currently playing media item
PlaylistManager() {
prepareMediaItems(); // Initialize media items
}
// Method to prepare and add media items to the list
void prepareMediaItems() {
_mediaItems.add(MediaItem(
videoId: "df18b398c85a48b2827ec694d96e0967",
title: "Big Buck Bunny",
thumbnailUrl: "https://d1z78r8i505acl.cloudfront.net/poster/w5wTtBSuo2Mv3.480.jpeg",
duration: 596,
otp: "example_otp",
playbackInfo: "example_playback_info"
));
// Add more media items as needed
}
// Method to get the list of media items
List<MediaItem> getMediaItems() {
return _mediaItems;
}
// Method to get media item at a specific index
MediaItem getMediaItemAtIndex(int index) {
return _mediaItems[index];
}
// Method to get the current media item
MediaItem getCurrentMediaItem() {
return _mediaItems[currentIndex];
}
// Method to play a media item at a specific index
MediaItem playMediaAtIndex(int index) {
currentIndex = index; // Set the current index
return _mediaItems[index]; // Return the media item at the index
}
// Method to play the next media item in the playlist
MediaItem playNext() {
currentIndex = (currentIndex + 1) % _mediaItems.length; // Move to the next index
return _mediaItems[currentIndex]; // Return the next media item
}
// Method to get the length of the playlist
int getPlaylistLength() {
return _mediaItems.length;
}
}
4. Handling Player Events
Add event listeners to manage player state changes, such as automatically playing the next video when the current one ends.
void _onEventChange(VdoPlayerController? controller) {
controller!.addListener(() {
VdoPlayerValue value = controller.value; // Get the current player value
setState(() {
vdoPlayerValue = value;
});
if (value.isEnded) { // Check if the video has ended
playNextMedia(); // Play the next media item
}
});
}
void playNextMedia() {
MediaItem mediaItem = playlistManager.playNext(); // Get the next media item
_controller?.load(mediaItem.getEmbedInfo()); // Load the next media item into player
}
Summary
This guide demonstrates how to integrate VdoCipher’s video playback features into a Flutter application. By setting up the vdocipher_flutter package, building a video player interface, and using a PlaylistManager
to handle media items and transitions, you can create a fully functional video streaming app. Handling player events ensures a seamless user experience, allowing for smooth transitions between videos. With the provided examples and steps, you can implement a robust video playback solution tailored to your needs.
For the complete implementation, you can find the source code on Github.
Feel free to customize and expand upon this implementation to suit your application's specific needs.
If you have any questions or need further assistance, please contact us at support@vdocipher.com