Implementing Picture in Picture Mode
Overview
Both Android (starting from Android 8.0, API level 26) and iOS (starting from iOS 9 for iPads and iOS 14 for iPhones) support Picture-in-Picture (PiP) mode. PiP allows users to watch video content in a small, floating window that remains visible while they navigate between apps or browse content on the main screen. This feature enhances multitasking by keeping the video playback active while the user interacts with other apps.
On both platforms, the PiP window can be resized and moved to different corners of the screen, ensuring a seamless experience across different use cases. The system determines the default placement of the PiP window, though users can reposition it.
Android
Declare picture-in-picture support
Register your video activity in your manifest by setting android:supportsPictureInPicture
to true
. Also, specify that your activity handles layout configuration changes as by setting android:configChanges
as mentioned below so that your activity doesn't relaunch when layout changes occur during PiP mode transitions.
<activity
android:name=".PlayerActivity"
android:configChanges="keyboardHidden|orientation|screenSize|smallestScreenSize|screenLayout"
android:supportsPictureInPicture="true">
Picture in picture implementation
To enter picture-in-picture mode, an activity must call enterPictureInPictureMode(). For example, the following code switches an activity to PiP mode when a the user clicks the home or recent button:
import android.app.PictureInPictureParams;
import android.os.Bundle;
import android.os.Build;
import androidx.annotation.RequiresApi;
import androidx.fragment.app.Fragment;
@RequiresApi(api = Build.VERSION_CODES.O)
@Override
protected void onUserLeaveHint() {
super.onUserLeaveHint();
// Switch to PiP mode if the user presses the home or recent button,
// Adding fragment check to ensure only the screen with player fragment can go into pip, remove this check
// if other screens can/should also go into pip mode
Fragment vdoPlayerFragment = getSupportFragmentManager().findFragmentByTag("VdoPlayerUIFragmentRN");
if (vdoPlayerFragment != null && vdoPlayerFragment.isVisible()) {
enterPictureInPictureMode(new PictureInPictureParams.Builder().build());
}
}
Handle UI during picture-in-picture
Use onPictureInPictureModeChanged
event based prop to handle UI during pip mode.
Refer example below to show/hide custom controls or other non-player related UI elements based on isInPictureInPictureMode
flag
import {VdoPlayerView} from 'vdocipher-rn-bridge';
const embedInfo = {
otp: 'some-otp',
playbackInfo: 'some-playbackInfo',
};
// in JSX
var isInPictureInPictureMode = this.state.isInPictureInPictureMode;
<VdoPlayerView
style={{height: 200, width: '100%'}}
embedInfo={embedInfo}
showNativeControls={false} // when using custom controls
onPictureInPictureModeChanged={this._onPictureInPictureModeChanged}
/>;
{
!isInPictureInPictureMode && (
<View style={styles.controls.container}>
<TouchableWithoutFeedback onPress={this._onPlayButtonTouch}>
<Icon name={showPlayIcon ? 'play' : 'pause'} size={30} color="#FFF" />
</TouchableWithoutFeedback>
<Text style={styles.controls.position}>
{digitalTime(Math.floor(this.state.position))}
</Text>
{this._renderSeekbar()}
<Text style={styles.controls.duration}>
{digitalTime(Math.floor(this.state.duration))}
</Text>
<TouchableWithoutFeedback onPress={this._toggleFullscreen}>
<MatIcon
name={isFullscreen ? 'fullscreen-exit' : 'fullscreen'}
style={styles.controls.fullscreen}
size={30}
color="#FFF"
/>
</TouchableWithoutFeedback>
</View>
);
}
_onPictureInPictureModeChanged = (pictureInPictureModeInfo: {isInPictureInPictureMode: boolean}) => {
this.setState({
isInPictureInPictureMode:
pictureInPictureModeInfo.isInPictureInPictureMode,
});
};
iOS
Picture in picture implementation
Enable Picture-in-Picture (PiP) mode in an iOS app using Xcode, as mentioned below:
Enable PiP capability: Open your project in Xcode and go to the "Signing & Capabilities" tab of your target. Click on the "+" button to add a new capability, then search for and add "Background Modes". In the list of background modes, check the option labeled "Audio, AirPlay, and Picture in Picture".
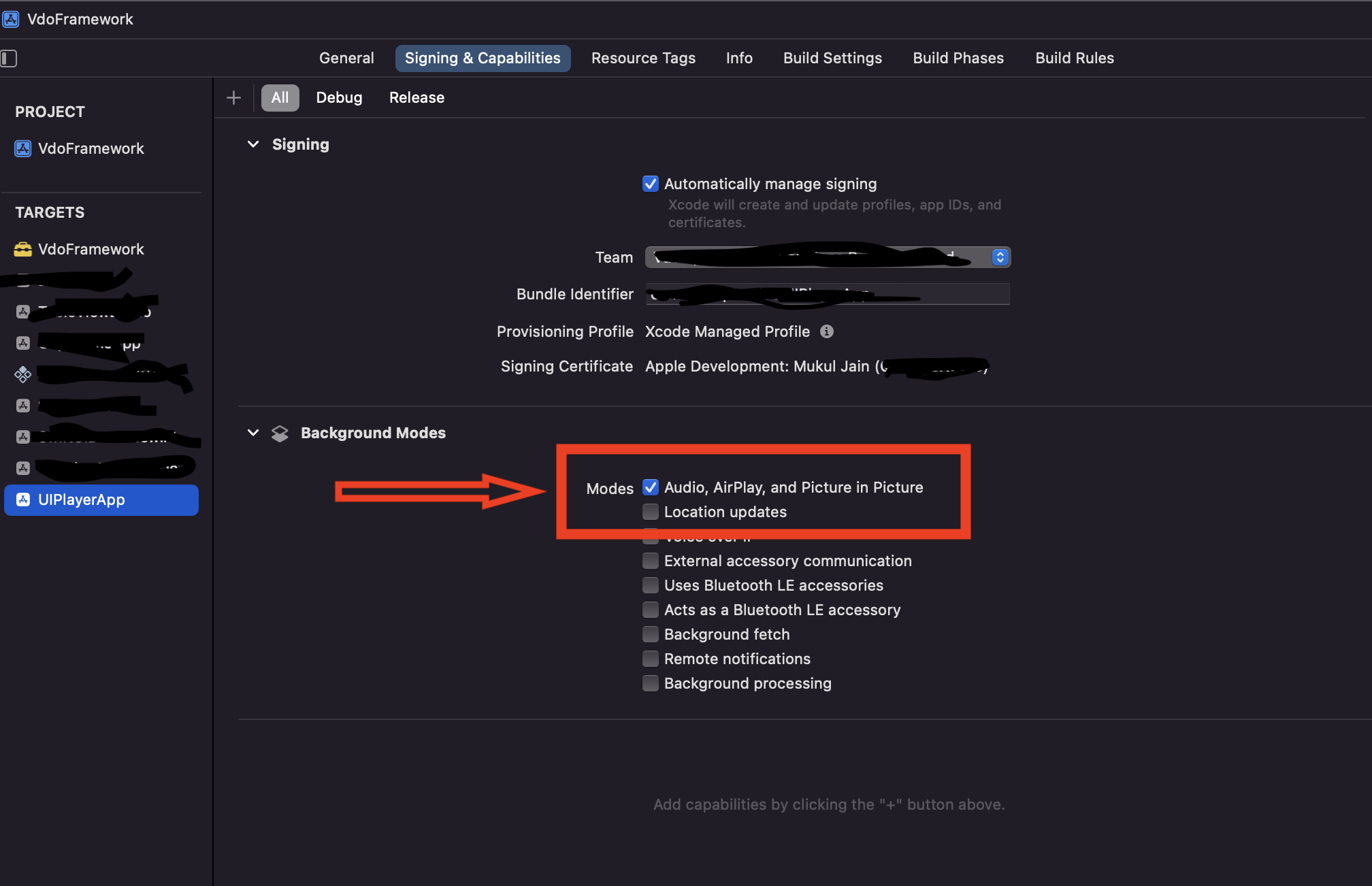